The Essential Elixir Cheatsheet for LeetCode Success
by Oskar Freye
A comprehensive guide to solving LeetCode problems efficiently with Elixir.
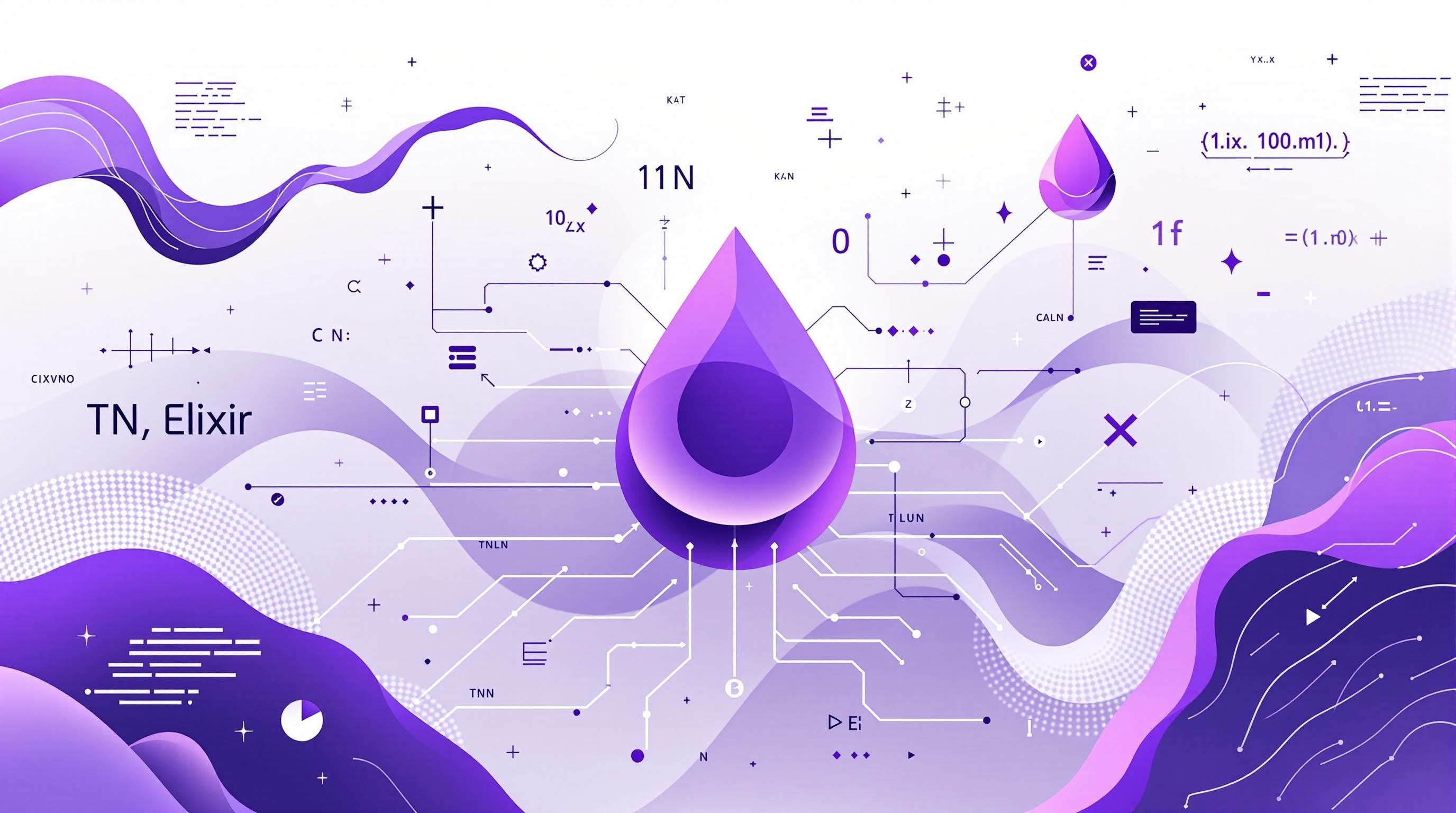
When tackling algorithmic challenges on LeetCode with Elixir, having the right tools and patterns at your disposal can make all the difference. Here’s your comprehensive guide to solving problems efficiently.
Core Data Structure Operations
List Operations
# Pattern matching fundamentals
[head | tail] = [1, 2, 3] # head = 1, tail = [2, 3]
# List construction
new_list = [element | existing_list] # [element | [2, 3]] = [1, 2, 3]
# List comprehension
for x <- list, condition, do: transformation
Map Operations
# Creation and access
map = %{key: value}
Map.get(map, :key, default)
Map.put(map, :key, value)
Map.update(map, :key, default, fn current -> new_value end)
MapSet Operations
set = MapSet.new()
MapSet.put(set, element)
MapSet.member?(set, element)
MapSet.intersection(set1, set2)
MapSet.difference(set1, set2)
Functional Building Blocks
Recursion Template
def process(input, acc \\ initial_value)
def process([], acc), do: acc
def process([head | tail], acc) do
process(tail, new_acc)
end
Stream Processing
Stream.iterate(initial, fn x -> next_value end)
Stream.unfold(initial, fn state -> {value, next_state} end)
Stream.zip(stream1, stream2)
Pattern Matching Templates
Multi-Clause Functions
def process(pattern1), do: result1
def process(pattern2), do: result2
def process(_), do: default_result
Guard Clauses
def process(x) when is_number(x) and x > 0
def process(x) when is_binary(x)
def process(x) when x in range
Common Helpers
String Processing
String.split(string, delimiter)
String.replace(string, pattern, replacement)
String.graphemes(string)
Number Processing
Integer.digits(number)
Integer.undigits(list)
div(a, b)
rem(a, b)
Collection Processing
Enum.reduce(collection, acc, reducer_fn)
Enum.flat_map(collection, mapper_fn)
Enum.group_by(collection, key_fn)
Common Transformations
List Transformations
# Accumulator transformation
def transform([], acc), do: acc
def transform([h | t], acc), do: transform(t, [transform_element(h) | acc])
# Non-accumulator transformation
def transform([]), do: []
def transform([h | t]), do: [transform_element(h) | transform(t)]
Tree Traversal Template
def traverse(nil), do: default_value
def traverse(node) do
# Process left, current node, right
process(node.value)
end